What are Docstrings in python? Docstrings in Python are special string literals used to document code within Python modules, functions, classes, and methods. They provide a way to explain the purpose, functionality, and usage of your code, making it easier for others (and yourself!) to understand what your code does.
What are Docstrings in python?
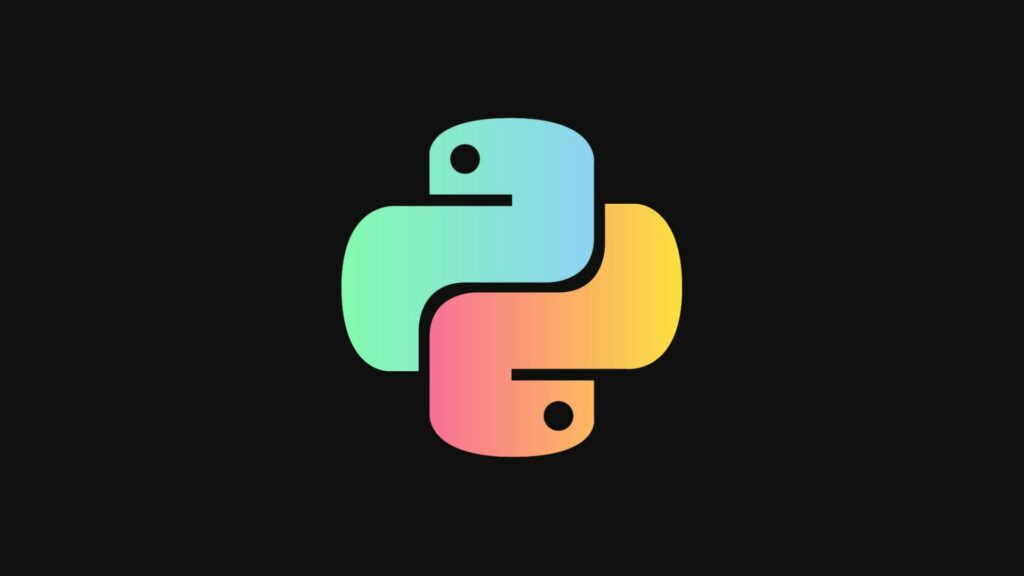
Here are some key points about docstrings:
Placement: Docstrings are typically placed as the first statement within a function, method, class, or module definition. #python docstrings
Triple quoted strings: Docstrings are written using triple quoted strings (either three single quotes ”’ or three double quotes “””). This distinguishes them from regular comments which use single or double quotes.
Content: A well-written docstring should include informative descriptions of:The purpose and functionality of the code block it describes.Parameters (arguments) accepted by the function or method, including their data types and meanings.Return value(s) produced by the function or method, including data types and descriptions.Any exceptions that the code might raise.Examples of how to use the code (optional).
Benefits of using Docstrings:
Improved code readability: Docstrings make code easier to understand for both yourself and others by providing clear explanations of what the code does.
Maintainability: Docstrings help maintain code by providing a reference point for understanding the code’s logic when revisiting it in the future or when someone else needs to modify it.
Automatic documentation generation: Tools like Sphinx can leverage docstrings to automatically generate comprehensive code documentation.
Here’s a simple example of a function with a docstring:
Python
def greet(name):
“””
This function greets the person by name.
Args:
name: The name of the person to greet (str).
Returns:
A string containing the greeting message (str).
“””
return f”Hello, {name}!”
# Call the function with a name
print(greet(“Alice”)) # Output: Hello, Alice!
I hope this explanation clarifies what docstrings are and how they can be helpful in Python programming!